When it comes to building dynamic and interactive web pages, sorting data is a crucial component. Sorting helps users find information quickly, and makes navigating large datasets more accessible. React, as a popular JavaScript library, has many tools that support sorting functionality. However, if you're looking for a more customized sorting solution, you may want to consider React Table Custom Sort.
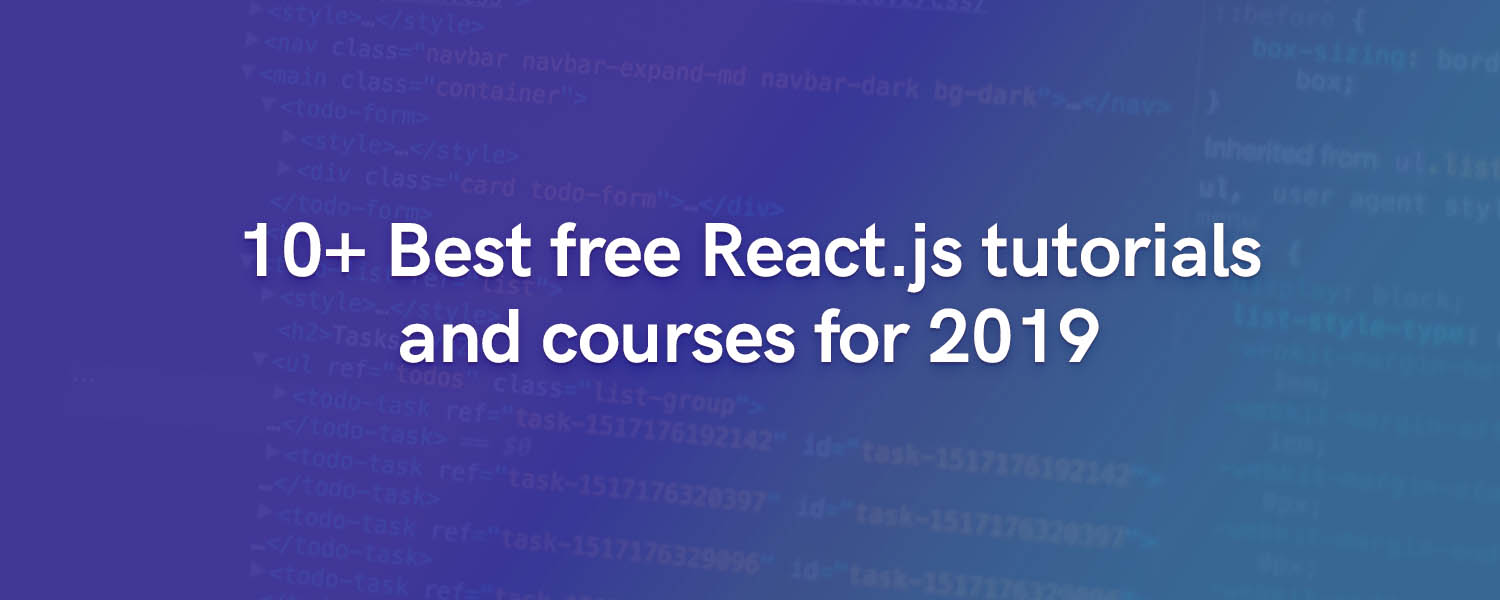
React Table is a library that simplifies the process of creating tables with data structure, formatting, and styling. The main goal of React Table is to offer a flexible approach to designing tables, allowing developers to create customized solutions without sacrificing performance. React Table Custom Sort extends this functionality by providing the ability to sort table data based on specific conditions that you define.
Installation
Before diving into React Table Custom Sort, you need to make sure you have React Table installed. You can install React Table using either npm or Yarn:
```
npm install react-table
```
```
yarn add react-table
```
Once you've installed React Table, you can install React Table Custom Sort using:
```
npm install react-table-addons
```
```
yarn add react-table-addons
```
After installing React Table and React Table Custom Sort, you need to import them into your project:
```javascript
import ReactTable from 'react-table';
import 'react-table/react-table.css';
import {withSort} from 'react-table-addons';
```
Using withSort Higher-Order Component
React Table Custom Sort is built on top of a Higher-Order Component (HOC) called withSort. The withSort HOC provides additional sorting functionality to React Table. To use the HOC, you need to wrap your React Table component with it:
```javascript
const CustomTable = withSort(ReactTable);
```
After wrapping the ReactTable component with withSort, you can pass custom sorting functions as props, which will then be used to sort the table's data. Here's an example:
```javascript
const sortFunction = (a, b) => a.age - b.age;
const columns = [{
Header: 'Name',
accessor: 'name'
}, {
Header: 'Age',
accessor: 'age',
sortMethod: sortFunction,
}];
const data = [{
name: 'Alice',
age: 28,
}, {
name: 'Bob',
age: 42,
}, {
name: 'Charlie',
age: 35,
}];
```
In this example, the sortFunction takes two parameters, a and b, which represent two items in the table's data object. The function returns the difference between the ages of the two items, which is then used to sort the data. The sortMethod property is added to the age column, which specifies that the sortFunction should be used to sort the column's data.
Using Multiple Custom Sort Functions
In some cases, you may need to sort data based on multiple conditions. React Table Custom Sort makes it easy to specify multiple custom sort functions. Here's an example:
```javascript
const columns = [{
Header: 'Name',
accessor: 'name',
sortMethod: (a, b) => a.name.localeCompare(b.name)
}, {
Header: 'Age',
accessor: 'age',
sortMethod: (a, b) => a.age - b.age,
}];
const data = [{
name: 'Alice',
age: 28,
}, {
name: 'Bob',
age: 42,
}, {
name: 'Charlie',
age: 35,
}];
```
In this example, the name column is sorted using the localeCompare method, which compares two strings and returns a negative value if the first string is before the second in alphabetical order. The age column is sorted using the same sortFunction as before.
Conclusion
React Table Custom Sort is a powerful tool for creating customized sorting solutions for tables in React. With just a few lines of code, you can sort data using custom functions based on the criteria that you define. The flexibility of React Table Custom Sort enables developers to create interactive, dynamic, and user-friendly tables that can handle large datasets. By using this functionality, you can improve user experience and offer an exceptional visual representation of your data.